Weather-related apps are becoming more and more necessary in our daily lives. They help choose which outdoor activities and trips to take, in addition to providing daily planning assistance and weather reports. Developers can learn a lot about data management, user interface design, and application programming interfaces (APIs) by creating a weather app.
This post will show how to build a simple yet strong weather application using JavaScript and the Weatherstack API. This course offers the required knowledge and code samples to help you start your path regardless of your level of expertise as a coder wishing to investigate new tools or a rookie developer trying to improve her skills.
Table of Contents
Overview of Weatherstack API
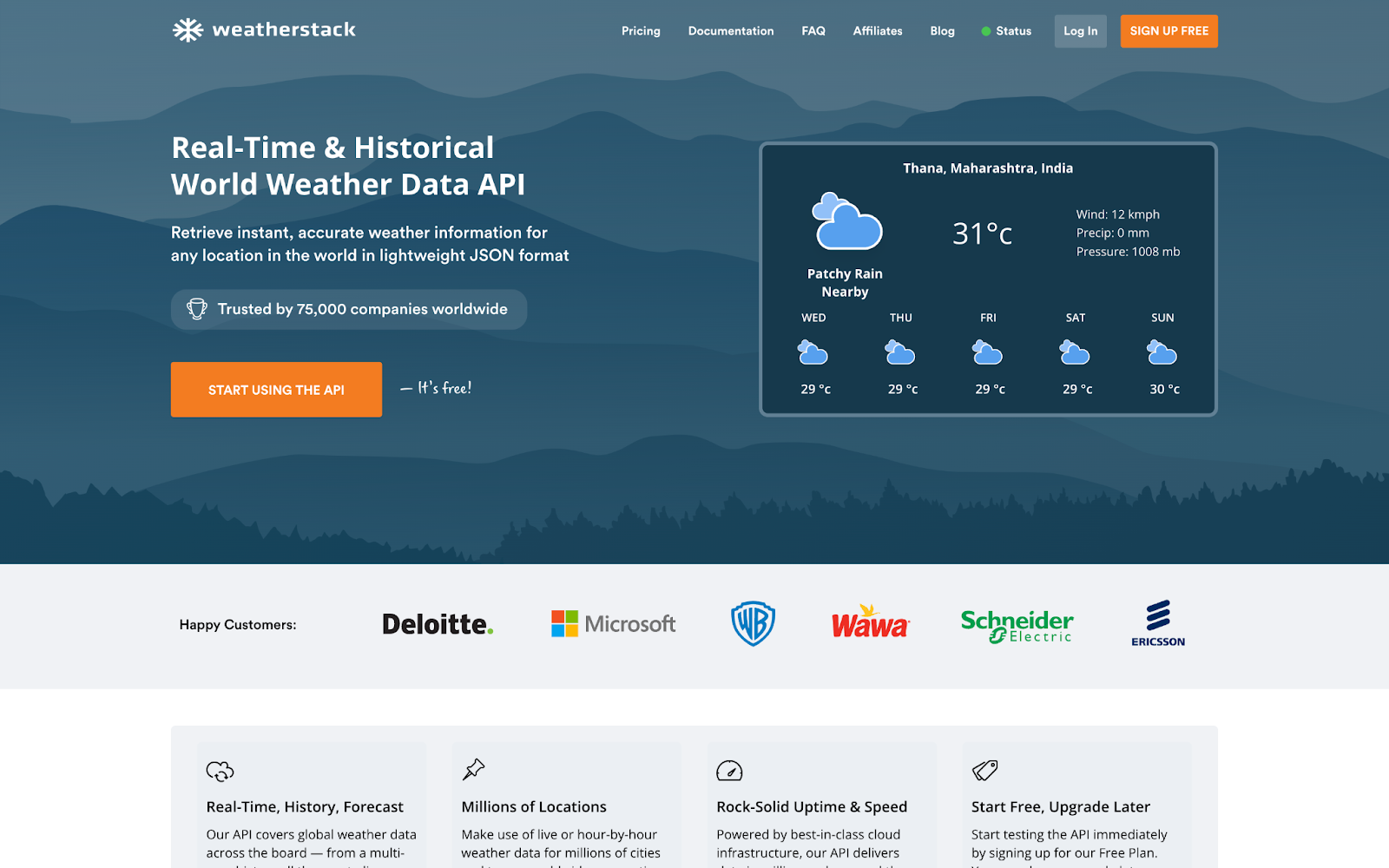
The Weatherstack API is a comprehensive and reliable weather data source for developers building weather-related applications. Weatherstack provides a variety of capabilities that may be smoothly incorporated into your projects, whether you’re building a simple weather widget or a sophisticated application that requires exact weather data.
This article gives a full review of the Weatherstack API, focusing on its core functionalities, features, and developer benefits.
Key Features of the Weatherstack API:
1. Real-Time Weather Data
The Weatherstack API’s ability to give you real-time weather data is one of its best benefits. This information has current weather conditions for any place in the world, including temperature, humidity, wind speed, and descriptions of the weather. Since the real-time data is changed often to make sure it is correct, it is perfect for users who need the latest weather information.
2. Historical Weather Data
Weatherstack also gives developers access to historical weather data, which lets them get information about the weather at different times in the past. This feature is especially helpful for tasks that need to look at trends, compare things, or understand the past. As an example, a vacation app might show users what the weather was like in a certain place on the same date last year.
3. Forecast Data
Forecasting the weather correctly is an important part of any weather program. Weatherstack’s API gives you accurate forecast info, like hourly and daily forecasts. With this feature, developers can make apps that help users plan their actions based on the weather that is going to happen.
4. Location-Based Weather Data
Weatherstack’s API supports queries by location, which can be specified in various ways, including:
- City Name: Query weather data for a specific city (e.g., “New York”).
- Coordinates: Use latitude and longitude to fetch weather data for a precise location.
- IP Address: Retrieve weather data based on the user’s IP address, which is useful for automatically detecting and displaying local weather.
5. Extensive coverage and data sources
Weatherstack collects information from a lot of reliable sources to give users news all over the world. So, your users will always be able to get correct weather information, no matter where they are. The API gives detailed weather reports for more than 200,000 cities and places, from big towns to remote areas.
6. Developer-Friendly Integration
It has a simple RESTful interface that makes it easy to add to any program. The Weatherstack API was made with developers in mind. Since the API uses standard HTTP requests, it can be used with any computer language that can send and read HTTP requests and JSON responses.
7. Scalability and Performance
The Weatherstack API is designed to handle a lot of calls, so it can be used for both small projects and big ones. The API can handle any number of calls your app makes every day, from a few hundred to millions.
8. Security and Compliance
When you use HTTPS, all the data sent between your app and the API is protected. This shows that Weatherstack takes security very seriously. So that sensitive user info stays safe and privacy rules are followed, this is a must.
9. Flexible Pricing Plans
Weatherstack has different pricing plans for different levels of use. There are free plans with restricted access to more advanced features, as well as enterprise plans made for applications that run a lot of data. Because of this, developers can start small and add more features as the app grows.
Getting Started: Setting Up Your JavaScript Weather App
Let’s get your weather app up and running by setting up your development setup.
Step 1: Sign Up for Weatherstack and Get an API Key
First, you’ll need to get your API key and create a Weatherstack account. You will be able to access the API and send requests for weather info using this key.
- Make a free account on Weatherstack.
- Go to your dashboard and find your API key after you’ve signed in.
Step 2: Setting Up Your Development Environment
A simple working environment with HTML, CSS, and JavaScript files is what you’ll need. You need to make a new directory for your project and add the following files to it.
- index.html
- style.css
- app.js
Fetching and Displaying Current Weather Data
Now that everything is set up, let’s get the latest weather information from the Weatherstack API and show it in your app.
Step 1: Basic HTML Structure
In your index.html file, create a simple structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather App</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<h1>Weather App</h1>
<div id="weather">
<p>Loading...</p>
</div>
</div>
<script src="app.js"></script>
</body>
</html>
Step 2: Styling with CSS
Now, add some simple styles to your style.css file to make the UI better:
body {
font-family: Arial, sans-serif;
background-color: #f7f7f7;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
text-align: center;
background-color: white;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
#weather {
font-size: 1.2rem;
margin-top: 20px;
}
Step 3: Fetching Weather Data with JavaScript
Let’s write the JavaScript code in app.js that will get the current weather information and show it.
const apiKey = 'YOUR_API_KEY';
const weatherContainer = document.getElementById('weather');
async function getWeather() {
try {
const response = await fetch(`http://api.weatherstack.com/current?access_key=${apiKey}&query=New York`);
const data = await response.json();
displayWeather(data);
} catch (error) {
weatherContainer.innerHTML = `<p>Error fetching weather data</p>`;
}
}
function displayWeather(data) {
if (data.error) {
weatherContainer.innerHTML = `<p>${data.error.info}</p>`;
} else {
const { temperature, weather_descriptions } = data.current;
const { location } = data.current;
weatherContainer.innerHTML = `
<h2>${location.name}, ${location.country}</h2>
<p>${weather_descriptions[0]}</p>
<p>${temperature}°C</p>
`;
}
}
getWeather();
It asks Weatherstack through an API for the current weather in New York and shows it in your app. Don’t forget to change “YOUR_API_KEY” to the API key you got earlier.
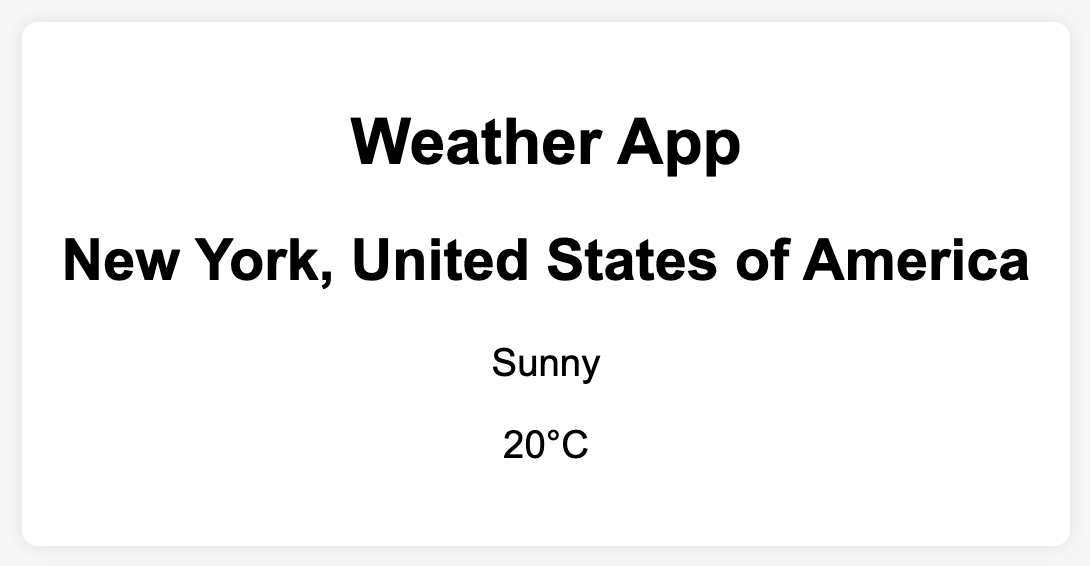
Adding Hourly and Daily Weather Forecasts
You can add hourly and daily weather reports to your app to make it more useful. With just a few changes to your API call, Weatherstack lets you get this information.
Step 1: Modify the API Request
In app.js, update the API request to include forecast data:
async function getWeather() {
try {
const response = await fetch(`http://api.weatherstack.com/forecast?access_key=${apiKey}&query=New York&forecast_days=1&hourly=1`);
const data = await response.json();
displayWeather(data);
} catch (error) {
weatherContainer.innerHTML = `<p>Error fetching weather data</p>`;
}
}
function displayWeather(data) {
if (data.error) {
weatherContainer.innerHTML = `<p>${data.error.info}</p>`;
} else {
const { location, current, forecast } = data;
weatherContainer.innerHTML = `
<h2>${location.name}, ${location.country}</h2>
<p>${current.weather_descriptions[0]}</p>
<p>${current.temperature}°C</p>
<h3>Forecast:</h3>
${displayForecast(forecast)}
`;
}
}
function displayForecast(forecast) {
const forecastHTML = [];
for (const date in forecast) {
const day = forecast[date];
forecastHTML.push(`
<div>
<h4>${date}</h4>
<p>Max Temp: ${day.maxtemp}°C</p>
<p>Min Temp: ${day.mintemp}°C</p>
<p>Average Temp: ${day.avgtemp}°C</p>
</div>
`);
}
return forecastHTML.join('');
}
getWeather();
Step 2: Displaying Forecast Data
This code will get the next day’s weather forecast and show it below the current weather information. You can make this even more unique by adding hourly reports. This is useful for apps that need to give a lot of information throughout the day.
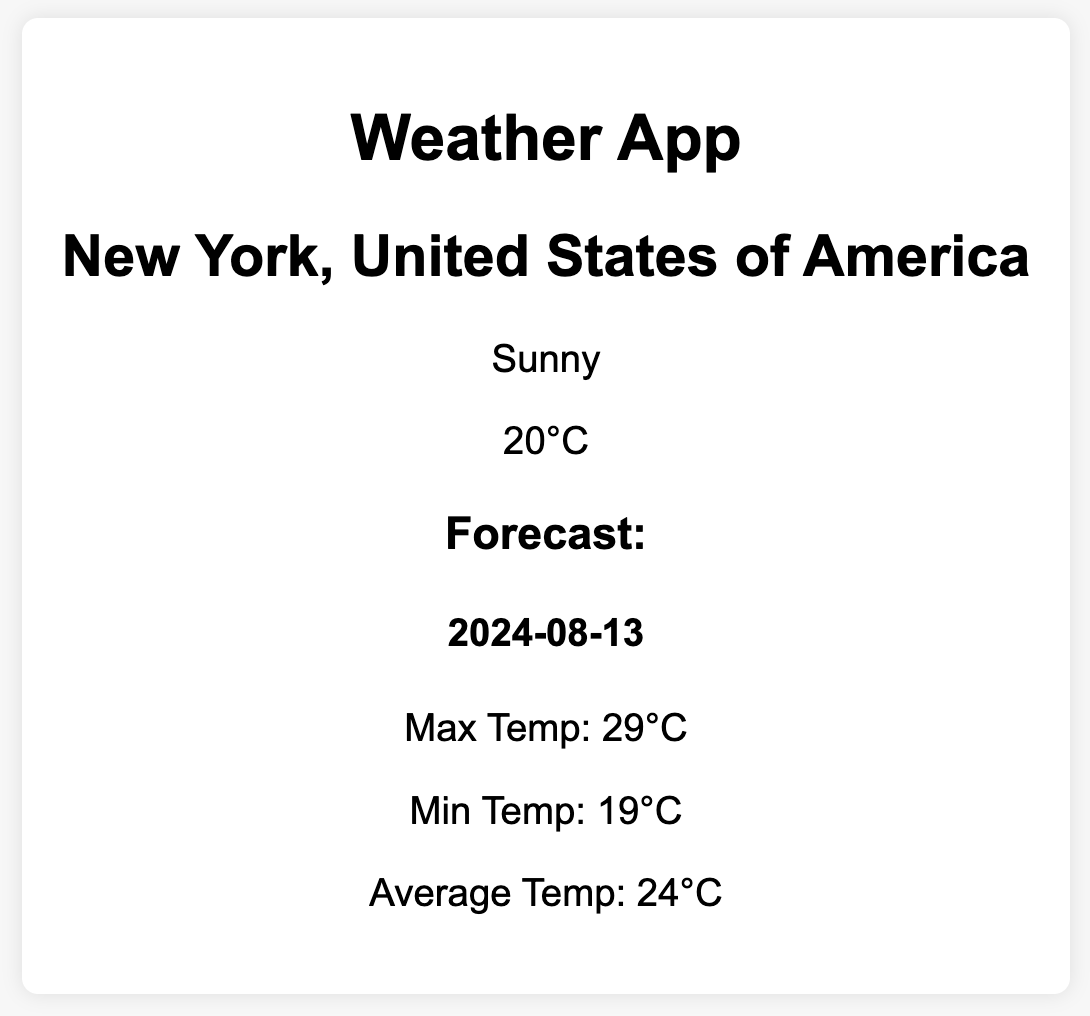
Addressing Common Developer Challenges
It can be hard to add a weather API like Weatherstack to your JavaScript app, especially if you are a developer who is new to adding APIs. For making a strong, effective, and easy-to-use weather app, it’s important to understand and deal with these problems. We’ll talk about some common problems developers might have and give you specific ways to fix them below.
1. Handling API Errors and Exceptions
Handling errors and exceptions is one of the most common challenges that come up when using APIs. There are many reasons why API requests might not work, including problems with the network, using the wrong API key, or passing in invalid query parameters. Users may see missing or incomplete data if these errors are not handled correctly, which can negatively impact the user experience.
Solutions:
Use try-catch blocks
If errors happen during API requests in JavaScript, you can use try-catch blocks to fix them. This method makes sure that your app doesn’t crash when an error happens, and you can instead show the user a useful error message.
async function getWeather() {
try {
const response = await fetch(`http://api.weatherstack.com/current?access_key=${apiKey}&query=New York`);
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
displayWeather(data);
} catch (error) {
weatherContainer.innerHTML = `<p>Error fetching weather data: ${error.message}</p>`;
}
}
Check for API error codes:
There are specific error codes that the Weatherstack API sends back when something goes wrong, like an invalid API key or too many requests. If these error codes show up in your app, it should handle them correctly. For example, you could tell the user to check their credentials if the API key isn’t correct.
function displayWeather(data) {
if (data.error) {
weatherContainer.innerHTML = `<p>Error: ${data.error.info}</p>`;
} else {
// Display weather data
}
}
Graceful Degradation:
Make sure your app can easily stop working when it can’t connect to the internet. If the API call fails, for instance, you could show a default message or tell the user to check their internet connection.
2. Managing and Securing API Keys
API keys are needed to make sure that requests to the Weatherstack API are legitimate, but if they are not handled properly, they can also be a security hole. If your API key is visible in client-side code, it could be used without your permission, which could cause you to go over your request limits or get charged for things you didn’t plan.
Solutions:
Environment Variables:
Never put your API keys straight into your JavaScript files in a production environment. Put them in environment variables on your server instead, and make sure only authorized people can view them.
// Example using Node.js and dotenv package
require('dotenv').config();
const apiKey = process.env.WEATHERSTACK_API_KEY;
Server-Side Proxy:
Set up an API proxy on the server to handle calls. Your API key will be kept safe on the server this way, and the client will only talk to the proxy. After sending the request to Weatherstack, the server sends the response back to the client.
// Example using Express.js as a proxy server
const express = require('express');
const fetch = require('node-fetch');
const app = express();
app.get('/weather', async (req, res) => {
const apiKey = process.env.WEATHERSTACK_API_KEY;
const response = await fetch(`http://api.weatherstack.com/current?access_key=${apiKey}&query=New York`);
const data = await response.json();
res.json(data);
});
app.listen(3000, () => console.log('Server running on port 3000'));
Rate Limiting:
To stop excessive API calls, implement rate limiting on your server. This keeps you from going over your API usage limits and stops people from abusing your API key.
3. Optimizing API Requests for Performance
Making a lot of API calls can slow down your app and cost you more, especially if you’re on a limited plan. To keep your app fast and cheap, you need to make sure that you optimize how and when you make API calls.
Solutions:
Debouncing Requests:
Use a debouncing method to cut down on the number of API requests your app makes when a user types something into a search box. Debouncing makes sure that the API request doesn’t happen until the user has stopped typing for a certain amount of time.
let debounceTimeout;
const debounce = (func, delay) => {
clearTimeout(debounceTimeout);
debounceTimeout = setTimeout(func, delay);
};
document.getElementById('search').addEventListener('input', () => {
debounce(() => {
getWeather(document.getElementById('search').value);
}, 500);
});
Caching Data:
Weather info should be cached on the client or server so that API requests aren’t made too often. You can use the cached data instead of making a new request if, say, the weather data hasn’t moved much in a certain amount of time (10 minutes).
let cachedData = null;
let lastFetchTime = null;
async function getWeather() {
const now = new Date();
if (cachedData && (now - lastFetchTime < 600000)) { // 10 minutes
return displayWeather(cachedData);
}
const response = await fetch(`http://api.weatherstack.com/current?access_key=${apiKey}&query=New York`);
const data = await response.json();
cachedData = data;
lastFetchTime = new Date();
displayWeather(data);
}
Reducing Data Payload:
Ask for only the information you need. You can tell the Weatherstack API which fields to send in the response. You can make the payload smaller and your application run faster by making sure the response only has the data it needs.
const response = await fetch(`http://api.weatherstack.com/current?access_key=${apiKey}&query=New York&units=m&fields=temperature,weather_descriptions`);
4. Ensuring Cross-Browser Compatibility
An important thing to keep in mind when making a JavaScript app is that it needs to work properly on all computers and devices. That is especially important for weather apps, which are often used on a variety of devices, such as computers, tablets, and phones.
Solutions:
Feature Detection:
You can use tools for feature detection like Modernizr to see if the user’s browser can handle certain features. This lets you set up fallbacks or other solutions for features that aren’t enabled.
if (Modernizr.geolocation) {
navigator.geolocation.getCurrentPosition(position => {
getWeather(position.coords.latitude, position.coords.longitude);
});
} else {
alert('Geolocation is not supported by your browser.');
}
Responsive Design:
Your app should be flexible, which means it should work on all screen sizes. To make sure users have the same experience on all screens, use CSS media queries and responsive design.
@media (max-width: 600px) {
.container {
width: 100%;
padding: 10px;
}
#weather {
font-size: 1rem;
}
}
Testing Across Browsers:
Check your app on Chrome, Firefox, Safari, and Edge, as well as other sites and devices, to see if there are any problems and fix them. For testing across browsers, tools like BrowserStack or Sauce Labs can be useful.
5. Enhancing User Experience with Robust UI/UX
One problem that many people face is making sure that their weather app not only works well but also is fun and easy to use. A well-designed UI/UX can make users happier and more likely to stick with your app.
Solutions:
Clear and Intuitive Design:
Users will find it easy to understand and use your app if the UI is clean and simple. To get weather information across quickly, use icons, colors, and fonts well.
.weather-icon {
width: 50px;
height: 50px;
margin: 0 auto;
}
.temperature {
font-size: 2rem;
color: #333;
}
.description {
font-size: 1.2rem;
color: #666;
}
User Feedback:
As users connect with the app, give them feedback in real time. For instance, show a spinning wheel while data is being fetched or a message when the weather data is complete.
<div id="loading" style="display:none;">
<p>Loading...</p>
</div>
async function getWeather() {
document.getElementById('loading').style.display = 'block';
try {
const response = await fetch(`http://api.weatherstack.com/current?access_key=${apiKey}&query=New York`);
const data = await response.json();
document.getElementById('loading').style.display = 'none';
displayWeather(data);
} catch (error) {
document.getElementById('loading').style.display = 'none';
weatherContainer.innerHTML = `<p>Error fetching weather data</p>`;
}
}
Accessibility:
Make sure that everyone can use your app, even people with disabilities. Make sure you use semantic HTML, give pictures alternative text, and make sure you can use a keyboard to move around in the app.
<button aria-label="Get current weather" onclick="getWeather()">Get Weather</button>
Enhancing the App with Additional Features
Once you’ve used JavaScript and the Weatherstack API to make a simple weather app, you can add several advanced features to make it more useful, give users a better experience, and make it more stable. You can add some extra features to your app by reading the details below, which also include helpful hints on how to do it.
1. Adding Geolocation to Automatically Fetch Weather Data
If you add geolocation to your weather app, it will automatically figure out where the user is and get the right weather information without them having to do anything. This function makes the user experience much better by giving them instant, localized weather information as soon as they open the app.
How to Implement Geolocation:
Use the Geolocation API:
The Geolocation API lets you get the user’s current location (latitude and longitude) and is supported by most modern browsers. After that, you can send these locations to the Weatherstack API to get information about the weather in that area.
function getWeatherByLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(position => {
const lat = position.coords.latitude;
const lon = position.coords.longitude;
fetchWeatherData(lat, lon);
}, error => {
console.error("Error fetching location: ", error);
// Provide fallback or error message to the user
});
} else {
console.error("Geolocation is not supported by this browser.");
}
}
function fetchWeatherData(lat, lon) {
const apiKey = 'YOUR_WEATHERSTACK_API_KEY';
const url = `http://api.weatherstack.com/current?access_key=${apiKey}&query=${lat},${lon}`;
fetch(url)
.then(response => response.json())
.then(data => displayWeather(data))
.catch(error => console.error("Error fetching weather data: ", error));
}
// Trigger the function to fetch weather by geolocation
getWeatherByLocation();
Fallback Mechanism:
Always have a backup plan in place in case geolocation isn’t available or the user doesn’t want to use it. You could, for instance, ask the user to enter their city manually or show the weather for the default location.
2. Incorporating Historical Weather Data
Historical weather data can be very useful for your app, especially for people who want to look at trends in past weather, compare today’s weather to weather from the past, or use past weather events to help them make decisions. With Weatherstack, you can get to past weather data that you can then show next to current weather data.
How to Implement Historical Data:
Requesting Historical Data:
If you include the date in your API request, you can get weather info from the past. You can use a date parameter in the style YYYY-MM-DD to ask Weatherstack for weather data from the past.
function fetchHistoricalWeatherData(city, date) {
const apiKey = 'YOUR_WEATHERSTACK_API_KEY';
const url = `http://api.weatherstack.com/historical?access_key=${apiKey}&query=${city}&historical_date=${date}`;
fetch(url)
.then(response => response.json())
.then(data => displayHistoricalWeather(data))
.catch(error => console.error("Error fetching historical weather data: ", error));
}
// Example usage: Fetching weather data for New York on July 1, 2023
fetchHistoricalWeatherData('New York', '2023-07-01');
Displaying Historical Data:
Make sure that your UI can show historical info well. You could compare the weather today to the same day last year, or you could give people a timeline view where they can scroll through weather data from the last week or month.
3. Integrating Hourly and Daily Forecasts
By adding hourly and daily forecasts to your weather app, you can help users plan their activities more accurately. While current weather reports give users immediate information, forecasts help users guess what the weather will be like in the future.
How to Implement Forecast Data:
Hourly Forecasts:
Get hourly weather data from the Weatherstack API and show it in a graph or format that can be scrolled. This is very helpful for people who are planning short-term activities.
function fetchHourlyForecast(city) {
const apiKey = 'YOUR_WEATHERSTACK_API_KEY';
const url = `http://api.weatherstack.com/forecast?access_key=${apiKey}&query=${city}&hourly=1`;
fetch(url)
.then(response => response.json())
.then(data => displayHourlyForecast(data))
.catch(error => console.error("Error fetching hourly forecast: ", error));
}
// Example usage: Fetching hourly forecast for New York
fetchHourlyForecast('New York');
Daily Forecasts:
You can use the API to get daily forecasts and show them in a way that is easy for people to understand, like a week view with daily highlights. To give people a quick overview, you can add icons, temperature ranges, and chances of rain or snow.
function fetchDailyForecast(city) {
const apiKey = 'YOUR_WEATHERSTACK_API_KEY';
const url = `http://api.weatherstack.com/forecast?access_key=${apiKey}&query=${city}&forecast_days=7`;
fetch(url)
.then(response => response.json())
.then(data => displayDailyForecast(data))
.catch(error => console.error("Error fetching daily forecast: ", error));
}
// Example usage: Fetching 7-day forecast for New York
fetchDailyForecast('New York');
4. Enhancing the User Interface with Advanced Features
For an interesting user experience, you need a well-designed user interface (UI). Adding advanced features to the user interface (UI) of your app can make it more engaging and look better.
UI Enhancements:
Custom Icons and Themes:
To represent various weather situations, use custom icons. You might want to add theme choices that let people choose between light and dark viewing modes.
<link rel="stylesheet" href="path-to-icons.css">
<div class="weather-icon sunny"></div>
Interactive Maps:
Add interactive maps that show what the weather is like in a bigger area. To see more weather information, users can zoom in and out or click on different places.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Leaflet Map Example</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet/dist/leaflet.css" />
<style>
#map {
height: 400px;
width: 100%;
}
</style>
</head>
<body>
<div id="map"></div>
<script src="https://unpkg.com/leaflet/dist/leaflet.js"></script>
<script>
var map = L.map('map').setView([51.505, -0.09], 13);
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
maxZoom: 19,
}).addTo(map);
L.marker([51.505, -0.09]).addTo(map)
.bindPopup('Current weather: Sunny, 22°C')
.openPopup();
</script>
</body>
</html>
Data Visualization:
You can see trends in weather data, like how temperatures change over the course of a day or a week, by adding charts and graphs. To make interactive visualizations, you can use libraries like Chart.js or D3.js.
Chart.js Library: Make sure that the Chart.js library is correctly included in your HTML file. You can include it via a CDN:
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
Canvas Element: Ensure that there is a corresponding <canvas>
element with the id="weatherChart"
in your HTML where the chart will be rendered:
<canvas id="weatherChart"></canvas>
Chart.js Version: The code snippet uses the syntax appropriate for Chart.js version 3.x or later. The y
axis configuration in the options
object is specific to this version. If you’re using an older version (2.x or earlier), the syntax for configuring the y
axis would be slightly different (scales.yAxes
instead of scales.y
).
JavaScript
// Example using Chart.js
var ctx = document.getElementById('weatherChart').getContext('2d');
var weatherChart = new Chart(ctx, {
type: 'line', // Line chart type
data: {
labels: ['12 AM', '3 AM', '6 AM', '9 AM', '12 PM', '3 PM', '6 PM', '9 PM'], // X-axis labels
datasets: [{
label: 'Temperature (°C)', // Label for the dataset
data: [15, 16, 18, 20, 22, 21, 19, 17], // Data points for the chart
borderColor: 'rgba(75, 192, 192, 1)', // Line color
borderWidth: 1 // Line width
}]
},
options: {
scales: {
y: {
beginAtZero: true // Y-axis begins at zero
}
}
}
});
5. Customizing the App’s UI for Better User Engagement
Personalization and customization let users make the app fit their needs, which can greatly increase user involvement. The app is easier to use and more appealing because it has custom alerts, themes, and translation.
Customization Features:
Custom Alerts:
Add a tool that lets users set their own weather alerts, like when it starts to rain, when the temperature drops too low, or when bad weather starts. You can get these tips through push notifications or see them in the app itself.
function setWeatherAlert(condition, message) {
if (currentWeather.condition === condition) {
alert(message);
}
}
setWeatherAlert('Rain', 'It’s going to rain today. Don’t forget your umbrella!');
Localization and Multi-Language Support:
Support multiple languages to reach people all over the world. Let people choose which language they want to use, and all weather information and user interface elements will be translated to that language.
const translations = {
en: {
temperature: 'Temperature',
humidity: 'Humidity',
wind: 'Wind Speed',
},
es: {
temperature: 'Temperatura',
humidity: 'Humedad',
wind: 'Velocidad del Viento',
}
};
function translate(language) {
document.getElementById('temperature-label').textContent = translations[language].temperature;
document.getElementById('humidity-label').textContent = translations[language].humidity;
document.getElementById('wind-label').textContent = translations[language].wind;
}
// Example usage: Translate to Spanish
translate('es');
6. Integrating Social Sharing and User Interaction
Make your app better by letting users interact with other app users or share weather information on social media sites. Features that let users share and interact with your app on social networks can bring more people to it and get them more involved.
Social Sharing:
Share Current Weather:
Put in place buttons that let people share the current weather on their social media sites with just one click. Add pre-written text that users can change before they share it.
<button onclick="shareWeather()">Share on Twitter</button>
<script>
function shareWeather() {
const weatherInfo = "It's currently sunny and 22°C in New York.";
const url = `https://twitter.com/intent/tweet?text=${encodeURIComponent(weatherInfo)}`;
window.open(url, '_blank');
}
</script>
User Interaction:
Commenting and Rating:
Make it possible for people to leave notes or rate how accurate the weather forecast is. This opinion could help make the app better and more focused on the user.
<div>
<textarea placeholder="Leave a comment"></textarea>
<button>Submit</button>
</div>
<div>
<label>Rate this forecast: </label>
<select>
<option>1</option>
<option>2</option>
<option>3</option>
<option>4</option>
<option>5</option>
</select>
</div>
You can turn a simple JavaScript weather app into a powerful tool that gives users detailed, personalized, and interesting weather information by adding these advanced features.
Conclusion
Building a simple JavaScript weather app with Weatherstack is a wonderful method to take advantage of accurate and dependable weather data. Following the methods indicated below will allow you to construct an application that not only offers real-time weather updates but also engages users with sophisticated features such as geolocation, historical data, and social sharing. Whether you’re a newbie or an experienced developer, Weatherstack’s user-friendly API provides the tools you need to create a sophisticated and feature-rich weather app.
Are you ready to bring your weather app ideas to life? Register on Weatherstack today and begin building your next weather-related project using one of the industry’s most trusted Weather APIs. Weatherstack offers a variety of plans, including a free tier, to accommodate every developer.
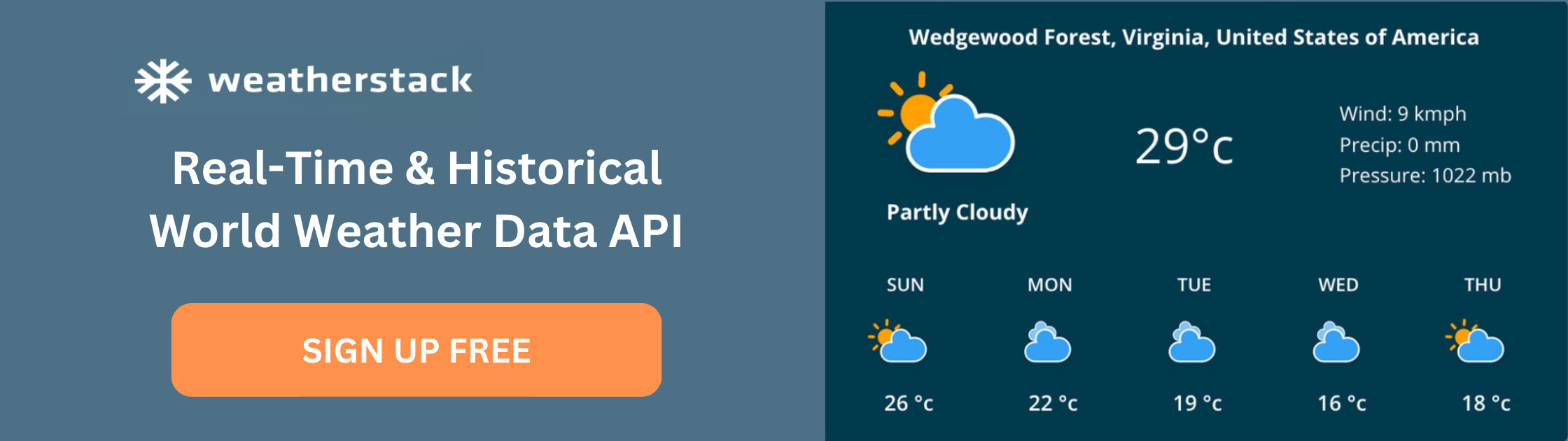
FAQs
How do I optimize my weather app for mobile devices?
Use CSS tools like Bootstrap or your own media queries to make sure that your app is responsive. Try the app out on different-sized screens and think about how to make it work best on mobile networks.
Can I customize the weather data returned by the Weatherstack API?
Yes, Weatherstack lets you change API requests so they include specific info like temperature, humidity, wind speed, and so on. Additionally, you can request data in various formats, such as JSON.
What do I need to start building a weather app using JavaScript and Weatherstack?
You’ll need to know the basics of JavaScript, get an API key from Weatherstack, and have a programming environment (like VS Code) ready to go. If you want to build the user experience, knowing HTML and CSS is also helpful.
Are there free and paid plans for weather APIs?
Yes, many weather APIs do have free tiers that only let you use the most basic functions. Paid plans give you access to more advanced tools, more data, and faster customer service.
Can I integrate other APIs with Weatherstack to enhance my weather app?
Yes, you can add other APIs, such as Google Maps for location data, Air Quality APIs for data about the environment, or even social media APIs for tracking weather. When you combine different APIs, you can make an app that does more and is more fun to use.
How can I deploy my weather app for public use?
You can use services like GitHub Pages, Netlify, or Heroku to publish your weather app after you’re done making it. You can put your app on these sites and share it with more people.
Can I integrate machine learning with Weatherstack to predict weather trends?
Yes, you can use machine learning models to look at Weatherstack data on the weather and guess what trends or odd events might happen in the future. This needs more data processing and model training, but it can make your app much more useful.