Today, there are many web services that businesses and developers use to fulfill their data needs. They obtain these web services from popular API marketplaces and use the data they provide to fulfill their data needs. Web services such as foreign exchange, IP geolocation, and web scraping are frequently used. While APIs like these are commonly used today, weather data is most frequently requested from external systems. Almost every business and developer today obtains data from a weather API for the weather dashboard that they present to their users.
Weather data has become a source that people can easily obtain with the developing technology. This data, which we see on almost many websites, is known as the fastest and easiest way to increase user satisfaction. Especially weather dashboard services developed with a popular weather API in the market easily allow businesses to get ahead of their competitors. In this context, in this article, we will first get to know a weather API that is preferred by popular businesses today to create great weather dashboards. Then, we will develop a weather dashboard with this API and then take a look at the future of these APIs. So let’s get started.
Table of Contents
The weatherstack API: The Best Weather Data API
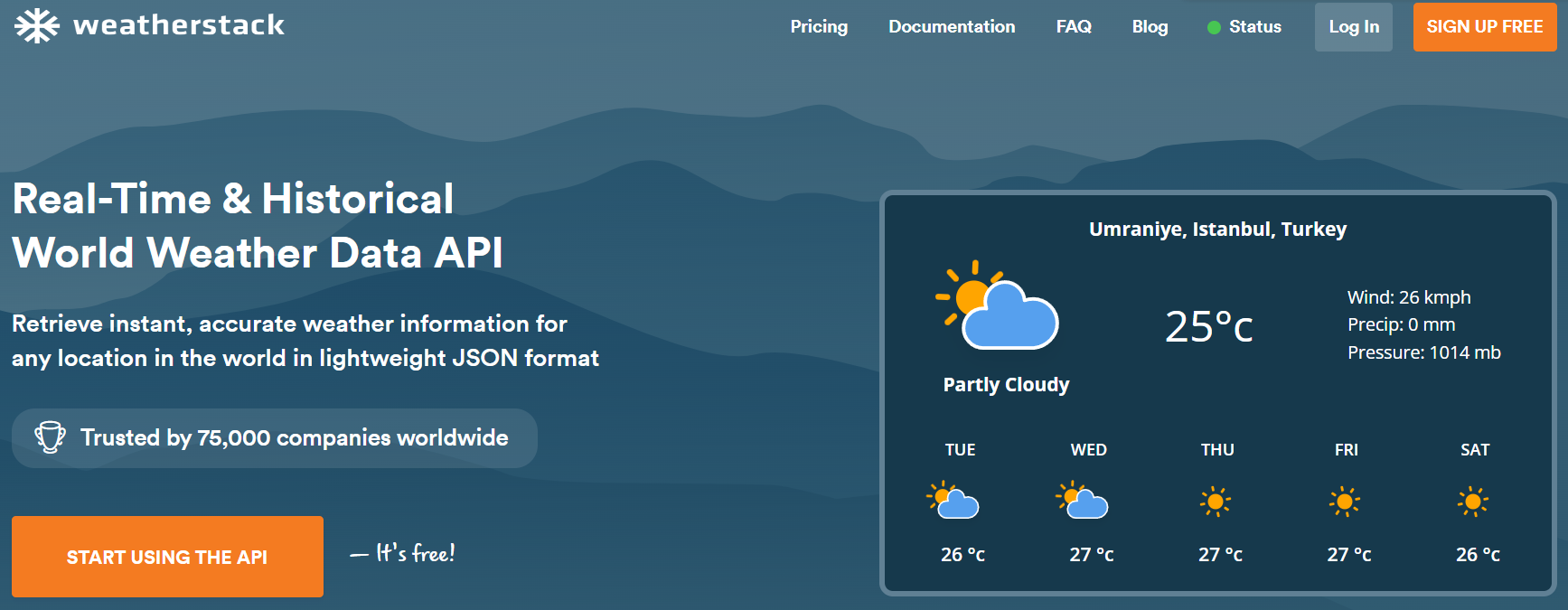
The weatherstack API is the best and most comprehensive global weather API on the market today. It provides live, accurate, and historical weather data for any location in the world in lightweight JSON format.
The weatherstack current weather API is one of the most reliable APIs on the market. It presents the weather data obtained from the official weather stations to its users. It also supports millions of locations around the world. Therefore, this API can be used safely by both local businesses and global businesses.
This API has 5 API features that it offers to its users. These:
- Current Weather: The most up-to-date current weather data for millions of locations.
- Historical Weather: Historical data from millions of locations up to 2008.
- Historical Time-Series: Historical time series data of millions of locations.
- Weather Forecast: Future weather forecast data of millions of locations.
- Location Lookup: Millions of location data.
One of the most preferred features of the weatherstack API is current weather. With this feature, the weather conditions provided by that desired location contain very detailed data, such as wind speed, wind degree, pressure, and humidity.
Discover how to build an API-driven weather app using weatherstack API.
Finally, one of the most important features that put the weatherstack API ahead of its competitors is its pricing policy. It has one free and three paid plans. The free plan offers users free access to up to 1,000 API requests per month. Paid plans start at $9.99 per month for 50,000 API requests.
Create a Current Weather Dashboard in a Web App
In this part, we will develop a web application using the weatherstack weather data API. In this web application, we will develop a weather dashboard using the current weather data endpoint of the weatherstack API.
Learn how to develop a global weather forecasting chatbot!
Before we start developing the application, we need an API key to use the weatherstack API. For this, let’s sign up for one of the affordable subscription plans it offers and get an API key.

Then, let’s open an HTML file in the file path we intend to develop the application and put the following codes in it.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather Dashboard</title>
<style>
*{
padding: 0px;
margin: 0px;
}
body
{
font-family: 'Roboto', sans-serif;
background: thistle;
}
.main{
width: 400px;
height: 200px;
margin: 150px auto;
background-color: #FFFFF0;
box-shadow: 0px 31px 35px -26px #080c21;
border-radius: 10px;
display: flex;
flex-direction: row;
}
.left{
margin-top: 20px;
padding: 15px;
}
.right{
margin-top: 54px;
margin-right: 5px;
margin-left: 25px;
}
.left-img{
width: 60px;
}
.right-img{
width: 180px;
border-radius: 20px;
}
.date{
font-size: 14px;
color: rgba(0,0,0,0.5);
font-weight: bold;
}
.city{
font-size: 21px;
color: rgba(0,0,0,0.7);
padding-top: 5px;
text-transform: uppercase;
font-weight: bold;
}
.temperature-section{
padding-top: 10px;
font-size: 61px;
color: rgba(0,0,0,0.9);
font-weight: 100;
}
.temperature{
font-size: 30px;
position: fixed;
padding-left: 12px;
padding-top: 9px;
}
.details {
font-size: 16px;
color: rgba(0, 0, 0, 0.7);
line-height: 1.5;
}
.details p span.bold-before-colon {
font-weight: bold;
}
</style>
</head>
<body>
<main class="main">
<div class="left">
<div id="date" class="date">
</div>
<div id="location" class="city"></div>
<div class="temperature-section">
<img id="weather-icon" alt="weather icon" class="left-img"/>
<span class="temperature" id="temperature"></span>
</div>
</div>
<div class="right">
<div class="details">
<p><span class="bold-before-colon" id="humidity-before">Humidity:</span> <span id="humidity"></span></p>
<p><span class="bold-before-colon" id="wind-before">Wind:</span> <span id="wind"></span></p>
<p><span class="bold-before-colon" id="pressure-before">Pressure:</span> <span id="pressure"></span></p>
<p><span class="bold-before-colon" id="uv-index-before">UV Index:</span> <span id="uv-index"></span></p>
</div>
</div>
</main>
<script>
// Weatherstack API URL
const apiUrl = "http://api.weatherstack.com/current?access_key=YOUR-API-KEY&query=New York";
// Fetch data from the API
fetch(apiUrl)
.then(response => response.json())
.then(data => {
console.log(data)
const current = data.current;
console.log(current.weather_icons[0])
// Update HTML elements with API data
document.getElementById("location").textContent = data.location.name;
document.getElementById("weather-icon").src = current.weather_icons[0];
document.getElementById("temperature").textContent = `${current.temperature}°C`;
document.getElementById("humidity").textContent = `Humidity: ${current.humidity}%`;
document.getElementById("wind").textContent = `Wind: ${current.wind_speed} m/s, ${current.wind_dir}`;
document.getElementById("pressure").textContent = `Pressure: ${current.pressure} hPa`;
document.getElementById("uv-index").textContent = `UV Index: ${current.uv_index}`;
// Format and update the date
const dateParts = data.location.localtime.split(' ')[0].split('-');
const formattedDate = `${dateParts[2]}/${dateParts[1]}/${dateParts[0]}`;
document.getElementById("date").textContent = formattedDate;
})
.catch(error => console.error("Error:", error));
</script>
</body>
</html>
With this code, we designed a unique dashboard by obtaining the instant weather conditions of the New York location with the weatherstack API. The result we get when we run the application is as follows:
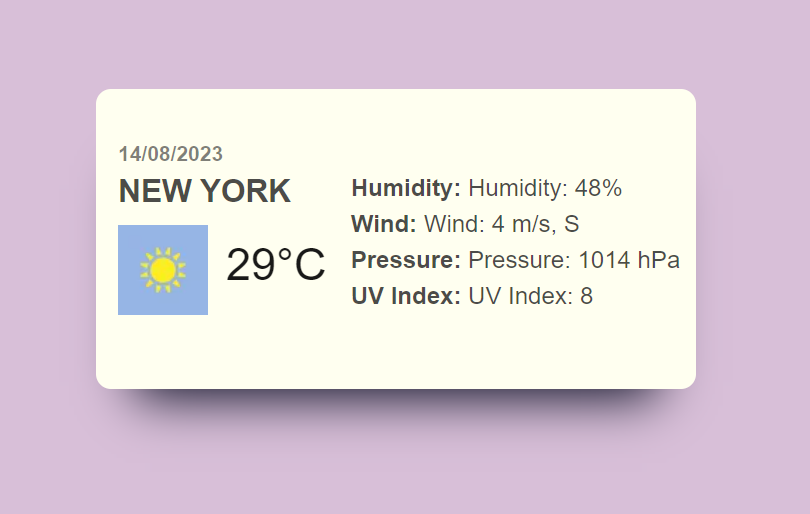
The Future of Weather Dashboard Services
The future of weather dashboard services will be shaped by becoming increasingly smarter and more personalized. Consequently, these services will make daily lives easier by providing customized forecasts according to users’ locations and needs. Moreover, the accuracy of the forecasts will increase, and the user experience will be improved thanks to artificial intelligence and machine learning algorithms.
Furthermore, weather dashboards will provide more comprehensive information by utilizing various data sources. Not only temperature and precipitation information, but also health-related data such as air quality, pollen status, and UV index will be provided to users. In this way, users will be able to make their daily plans more consciously and healthily.
In the future, these dashboards will integrate with smart home systems and wearable technologies to provide more proactive services. For example, automatic jacket-wearing suggestions or sunscreen-use reminders before going out will be possible. Such innovations will further increase the importance of weather dashboard services in our lives.
Conclusion
As a result, the increasing importance and use of weather dashboard services make it easier to act more consciously and plan in daily life. People can better organize their activities depending on weather conditions and take precautions against adverse weather conditions. At this point, weatherstack’s provision of live weather data makes a significant difference. Weatherstack increases the reliability and accuracy of weather dashboards with the instant and accurate information it provides, allowing users to access the most up-to-date information quickly and easily. Thus, users who have more detailed and instant information about the weather have the opportunity to manage their daily lives and plans more effectively.
Try our best current weather data provider and offer your users a unique experience!
FAQs
Q: How Do Weather Dashboard Services Increase User Satisfaction?
A: Weather dashboards provide users with instant and accurate weather information, allowing them to better plan their daily plans. They enhance the user experience by providing personalized forecasts and recommendations based on weather conditions. They also help users protect their health and safety with additional information such as air quality and UV index.
Q: Is the weatherstack API a Free Weather API?
A: Yes, it is. The weatherstack real-time weather data API offers its users a free plan for up to 1,000 API calls per month.
Q: Can I Create Severe Weather Alerts with the weatherstack Weather API’s Data?
A: Yes, you can. You can create weather alerts thanks to the detailed data that the weatherstack API provides to its users.
Q: How Many Local Weather Models Does the weatherstack API Provide?
A: The weatherstack API has an extensive network of locations supporting millions of locations around the world.
Q: Does the weatherstack API Provide Weather Forecasts?
A: Yes, it does. It has a unique endpoint where it offers weather forecasts. Also, it can present hourly forecasts.
Read: How to Create a Great Weather Dashboard With a Weather API – Part 2 (Historical Weather)
Read: How to Create a Great Weather Dashboard With a Weather API – Part 3 (Weather Forecast)
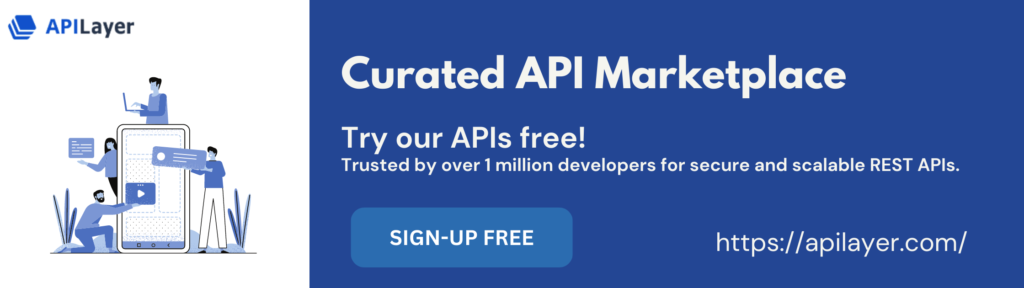