Weather data is delivered to people in many ways today. Newspapers, news channels, weather apps, and more. The use and popularity of weather applications are more than others. Today, many smartphones now come with a weather app installed. Popular weather apps like Weather Underground are excellent services that provide us with unique information such as current weather, up-to-date weather forecast, and historical weather conditions.
Well, have you ever thought about how a weather application provides us with live, historical, and even up-to-date weather forecast data? A weather application typically provides its users with weather conditions data using a weather API. In this article, we will first touch on the concept of a weather API. Then we will introduce today’s most popular weather API and develop a user-friendly weather app with this API.
Table of Contents
What Is a Weather API?
A Weather API (Application Programming Interface) is a web service that provide weather data to developers and businesses. These APIs provide their users with data from official weather sources. Moreover, weather APIs usually provide data to their users with API keys.
There are some features that a good weather API should have. Some of those:
- Rich Weather Data: A good weather API should provide its users with basic weather information (temperature, humidity, wind speed, precipitation, sunny/cloudy, etc.).
- Up-to-date Weather Data: This API should provide the most up-to-date weather data possible.
- Forecasts and Future Data: Another feature that makes a weather API popular is that it provides forecast data. These forecasts can help users plan or make decisions based on the weather.
- Wide Location Support: A good weather API should provide rich location support to its users. Many users in the global age should also be able to use this API.
- Stability and Reliability: It is very important for a weather API to have high uptime and the accuracy of the data it provides.
Some Popular Weather APIs
Today there are many weather APIs that provide weather data. Among these APIs, some of the best weather APIs to use in a simple weather app are as follows:
- The weatherstack API
- AccuWeather API
- OpenWeatherMap API
- Open-Meteo API
- Visual Crossing Weather API
- Weather API
- Storm Glass API
- World Weather Online API
The weatherstack API: The Best Official Weather API
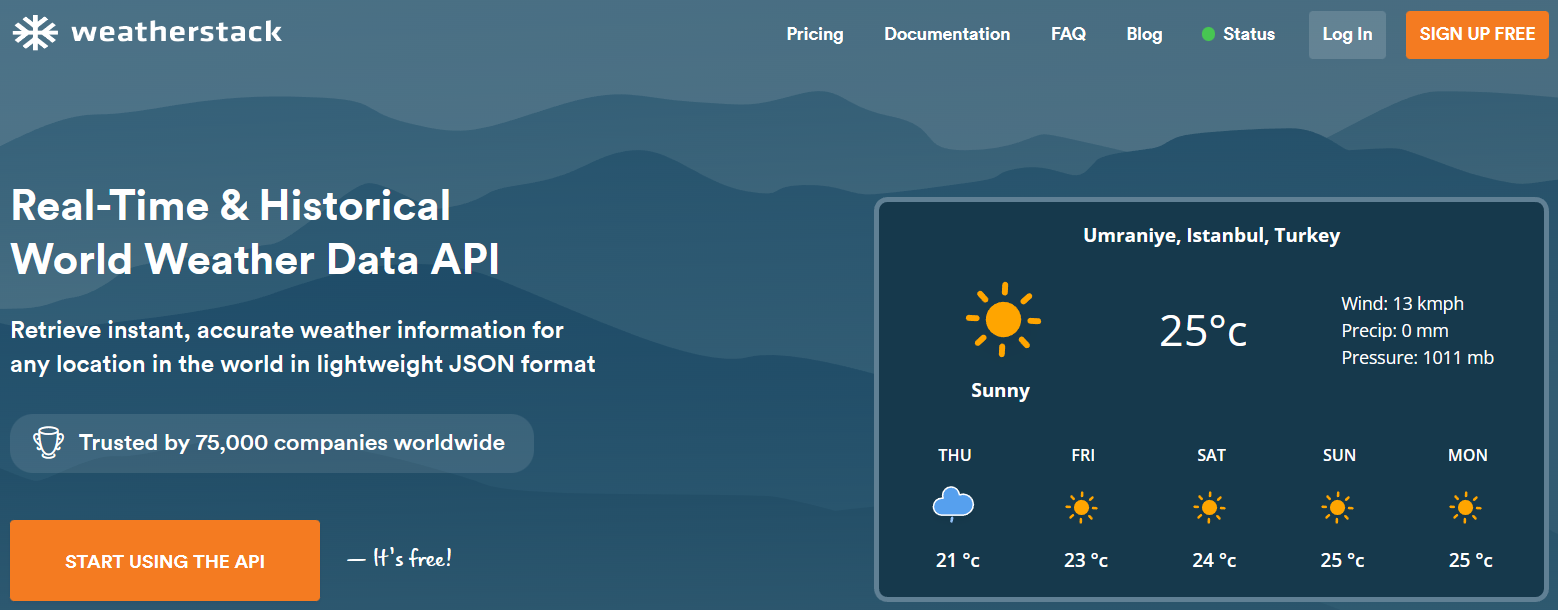
The weatherstack API is one of the most sophisticated and comprehensive weather APIs on the internet. They actively serve more than 75,000 companies. Among these companies, there are companies with global users, such as Microsoft, Deloitte, and Ericsson.
The weatherstack API, which is also used by today’s best weather apps, provides its users with the most up-to-date weather data. It also provides real-time weather data as well as historical weather data and weather forecasts. Moreover, this API also supports millions of locations around the world. In this way, it serves thousands of global customers today.
This API provides its users with highly accurate data by obtaining its data from reliable and powerful data providers. It is also a very simple API to use, and developers can integrate this API into any programming language in just a few steps.
Learn the importance and benefits of historical weather data!
What Are the Subscription Plans of the weatherstack API?
The weatherstack API provides its users with a very innovative pricing policy. It has multiple subscription plans.
The first of these plans is the free plan. This API offers 250 requests per month without asking for any credit card information from its users with the free plan.
Apart from the free plan, this API has three paid plans. These plans start at $9.99 per month for 50,000 requests and go up to $99.99 per month for 1,000,000 requests.
Furthermore, this API also has a custom subscription plan that it offers to its users for enterprise use. For this, users can contact the APILayer easily.
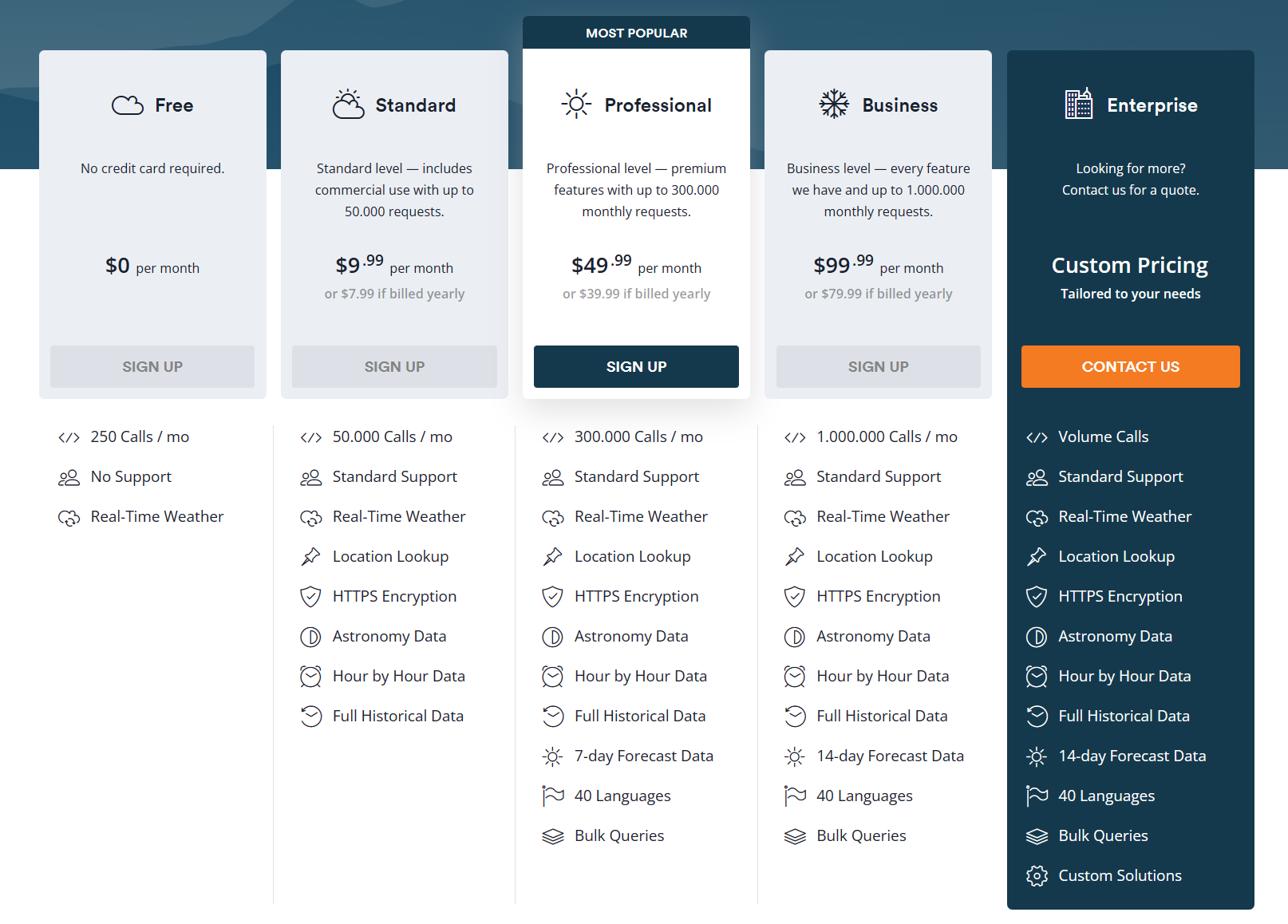
How to Create a Weather App With the weatherstack API?
In this section, we will develop a weather web application whose weatherstack API provides us with the current weather data in the location we want. We will use HTML, CSS, JavaScript, and Bootstrap while developing this application. We will also develop the application in a single HTML file.
Before we start developing the application, we need an API key to get the current weather data from the weatherstack API. To get this API key, let’s sign up for one of the affordable subscription plans offered by the weatherstack API.

After registration, we can get the API key and can start developing our application. Let’s open a file named ‘current-weather-app-with-the-weatherstack.html’ in the file path, where we will develop the application and put the following codes into this file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather App</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<style>
body {
padding: 20px;
}
.weather-card {
max-width: 400px;
margin: 0 auto;
background-color: #f7f7f7;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
.weather-icon {
max-width: 100px;
margin: 0 auto;
display: block;
}
</style>
</head>
<body>
<div class="container">
<div class="weather-card">
<h1 class="text-center">Current Weather</h1>
<form id="location-form">
<div class="form-group">
<label for="location-input">Location:</label>
<input type="text" class="form-control" id="location-input" placeholder="Ex: New York">
</div>
<button type="submit" class="btn btn-primary">Search</button>
</form>
<div id="weather-info" class="mt-4"></div>
</div>
</div>
<script>
const form = document.getElementById('location-form');
const input = document.getElementById('location-input');
const weatherInfo = document.getElementById('weather-info');
form.addEventListener('submit', (e) => {
e.preventDefault();
const location = input.value.trim();
// variable called API Key
const APIKey = 'YOUR-API-KEY';
fetch(`https://api.weatherstack.com/current?access_key=${APIKey}&query=${encodeURIComponent(location)}`)
.then(response => response.json())
.then(data => {
renderWeatherData(data);
})
.catch(error => {
console.log(error);
weatherInfo.innerHTML = '<p class="text-danger">Please try again!</p>';
});
input.value = '';
});
function renderWeatherData(data) {
const location = data.location;
const currentWeather = data.current;
const html = `
<h2 class="text-center">${location.name}, ${location.country}</h2>
<div class="text-center">
<img src="${currentWeather.weather_icons[0]}" alt="${currentWeather.weather_descriptions[0]}" class="weather-icon">
<p>${currentWeather.weather_descriptions[0]}</p>
<p>Temperature: ${currentWeather.temperature}°C</p>
<p>Feels Like: ${currentWeather.feelslike}°C</p>
<p>Wind Speed: ${currentWeather.wind_speed} km/s</p>
<p>Humidity: ${currentWeather.humidity}%</p>
<p>UV Index: ${currentWeather.uv_index}</p>
<p>Observation Time: ${currentWeather.observation_time}</p>
</div>
`;
weatherInfo.innerHTML = html;
}
</script>
</body>
</html>
This piece of code will obtain the current weather information of the location that the user enters from the weatherstack API via the fetch API, and print it on the screen. Before running the application, let’s write our own API key to the ‘APIKey’ variable and run the application.
After running the application, the following page will welcome us.
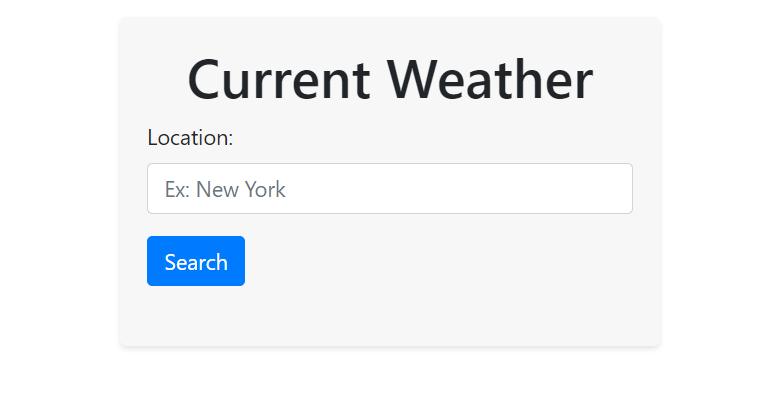
After typing ‘Berlin’ in the location field on this weather page and pressing the ‘Search’ button, the current weather data presented to us by the application will be as follows.
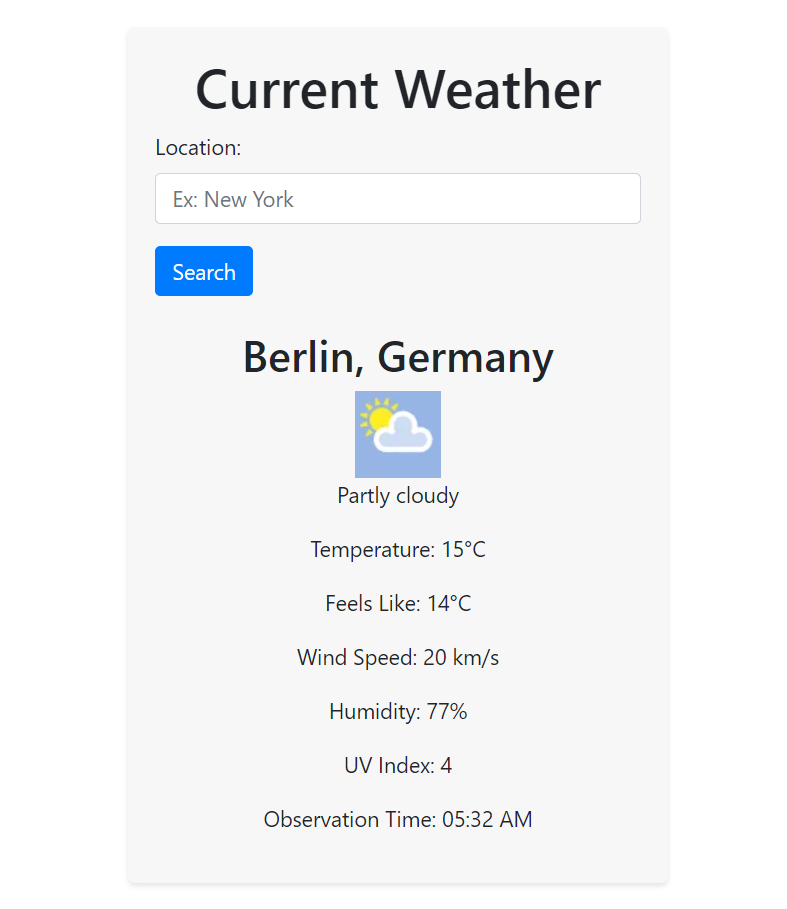
Conclusion
To sum up, nowadays, there are many platforms where people can get weather data. Among these platforms, weather apps are the fastest and most effective. Today, multiple weather apps serve both on websites and mobile applications. These weather apps usually use weather APIs that provide comprehensive data and provide users with live, historical, and weather forecast data.
FAQs
Q: What are Some Popular Use Cases of the Weather Data?
A: Weather data is frequently used in many applications and platforms today. Some of the use cases of weather data are as follows:
- Real-Time and Historical Weather App
- Weather Forecast App
- Weather News App
- Weather Channel
- Severe Weather Alerts
Q: Do I Need an API Key to Get the Current Weather data From the weatherstack API?
A: Yes, you do. You need an API key to use multiple services offered under the weatherstack API. In order to obtain data from the weatherstack API, first choose one of the flexible subscription plans that this API provides to you, and then register. After registration, your API key will be generated.
Q: How Should a Weather API that Using in a Weather App?
A: There are some key features that a weather API used in weather applications should have. Some of the features that this API should provide are as follows:
- Rich Weather Data
- Current Data
- Forecasts and Future Data
- Wide Location Support
- Stability and Reliability
- Powerful Documentation
Q: What are the Most Popular Weather APIs Used in Weather Apps?
A: There are many comprehensive weather APIs available on the Internet for developers and businesses to choose from. Among these weather APIs, the most popular weather APIs used in applications are as follows:
- The weatherstack API
- AccuWeather API
- OpenWeatherMap API
- Open-Meteo API
- Visual Crossing Weather API
Q: Is the weatherstack API a Global Weather Data Provider?
A: Yes, it is. The weatherstack API provides weather data for millions of locations around the world. This API offers the most up-to-date and fastest weather data in all supported locations.