Recently, we see that weather data is used in almost many applications today. News sites, mobile applications, e-commerce sites, and many more platforms are examples of this. It is possible to say that platforms that present global weather information on a weather dashboard touch more users. Recently, we have seen that historical weather data is presented to users with a historical weather API, especially on many platforms.
A weather dashboard, which also provides historical weather data, has recently been used on the homepage of many platforms. Many mobile applications even offer their users as widgets. The importance of historical weather data is huge for businesses and developers. In this article, we will examine the importance and use cases of historical weather data. But first, let’s list the most popular historical weather APIs from which businesses and developers can obtain historical weather data.
Table of Contents
What are the Some Popular Historical Data Providers?
The increase in the importance and use of historical weather data has also increased the number of APIs serving in this field. Today, it is possible to find a historical weather API suitable for almost every project that businesses and developers can prefer in the market.
Among the many historical weather APIs on the market, the most important is the weatherstack API. This API provides historical weather data up to 2008 for millions of locations. For a specific location, it can provide weather data with a particular date, while also providing weather data relative to a date range. In addition, it offers developers the opportunity to customize their queries with many optional parameters. Thanks to all these features, it is preferred by giant companies such as Microsoft, Warner Bros, and Ericsson today.
Compare the 6 best weather APIs of 2023!
Today, apart from the weatherstack API, there are many popular APIs that provide historical weather data. Some of them are as follows:
- OpenWeatherMap API
- Aeris Weather API
- Tomorrow.io API
What is the Importance of the Historical Weather Data?
Historical weather data is of great importance in terms of understanding past weather conditions in various sectors and areas, making future plans, and managing risks. This data helps businesses gain a more comprehensive view of current and future weather conditions, while also serving as a critical tool in shaping their sustainability efforts and risk management strategies.
Some popular uses for the importance of historical weather data are as follows:
- Climate Change Analysis: The most popular use case of historical weather data is its use in long-term climate change analysis. This data includes weather conditions such as natural events, temperature, precipitation, and wind that have occurred in the past years. These analyses help businesses and analysts understand long-term climate trends and predict future climate changes.
- Agriculture: The agricultural sector is an area where weather conditions have a major impact on product productivity and quality. Historical weather data is used to plan future farming seasons, optimize irrigation schedules, and determine crop harvest timings by analyzing weather conditions in past years.
- Energy Management and Planning: The efficiency of renewable energy sources such as wind and solar energy depends on weather conditions. Historical weather data is used to evaluate the performance of these energy sources and to optimize energy production. This data is also used to forecast electricity demand and plan energy supply.
- Travel and Tourism: Weather is one of the most important factors when making holiday plans. Historical weather data helps tourists make their travel plans by analyzing the weather conditions of a particular region for a particular period.
Create a Striking Historical Weather Dashboard in a Web App
In this part, we will develop a very useful weather dashboard that provides historical data using the weatherstack API. We will develop the application using HTML, CSS, and JavaScript.
Learn how to visualize global weather data in Python.
Before we start developing the application, we need to obtain the API key we need to use the weatherstack API. For this, let’s sign up for one of the paid subscription plans offered by the weatherstack API and get an API key.
After obtaining the API key, let’s create an HTML file and put the following code in this file:
<!DOCTYPE html>
<html>
<head>
<title>Historical Weather Dashboard</title>
<style>
body {
font-family: "Montserrat", sans-serif;
text-align: center;
padding: 100px;
}
.weather {
perspective: 1000px;
}
.weather_dashboard {
width: 340px;
margin: 0 auto 1%;
box-shadow: 0px 0px 10px 0px rgba(0, 0, 0, 0.3);
border-radius: 10px;
}
.dashboard-header {
text-align: center;
position: relative;
overflow: hidden;
height: 215px;
padding-bottom: 40px;
background-color: aliceblue;
}
.header-temperature {
position: absolute;
z-index: 200;
text-align: center;
font-size: 42px;
left: 50%;
transform: translateY(1px) translateX(-50%);
text-shadow: 1px 1.732px 3px rgba(0, 0, 0, 0.2);
}
.hour {
position: absolute;
z-index: 200;
text-align: center;
font-size: 22px;
font-weight: light;
left: 50%;
transform: translateY(-48px) translateX(-50%);
text-shadow: 1px 1.732px 3px rgba(0, 0, 0, 0.2);
}
.main-content {
position: relative;
padding: 10px 0;
text-align: left;
left: 0;
width: 100%;
bottom: 0;
transition: bottom 0.3s;
background: white;
box-shadow: 0px -3px 10px 0px rgba(0, 0, 0, 0.2);
display: table;
}
.weather-column {
padding: 0 0 20px;
display: table-cell;
text-align: center;
opacity: 0.5;
transition: all 0.3s;
cursor: pointer;
}
.is-active {
opacity: 1;
transition: all 0.3s;
}
.day {
text-transform: uppercase;
font-weight: bold;
font-size: 20px;
margin: 10px 0 0;
}
.temperature {
margin: 5px 0 0;
}
.weather-desc{
position: fixed;
margin-top: -58px;
font-size: 22px;
transform: translateY(160px) translateX(-50%);
text-shadow: 1px 1.732px 3px rgba(0, 0, 0, 0.2);
left: 50%;
}
.location{
font-size: 30px;
}
</style>
</head>
<body>
<div class="weather">
<section class="weather_dashboard">
<article>
<header class="dashboard-header">
<p id="location" class="location" ></p>
<p id="weather_description" class="weather-desc"></p>
<h1 id="temperature" class="header-temperature"></h1>
<p id="hour" class="hour"></p>
</header>
<main class="main-content">
</main>
</article>
</section>
</div>
<script>
const apiUrl = "http://api.weatherstack.com/historical?access_key=YOUR-API-KEY&query=New York&historical_date_start=2023-08-10&historical_date_end=2023-08-14";
fetch(apiUrl)
.then(response => response.json())
.then(data => {
const current = data.current;
// Update header elements with API data
document.getElementById("hour").textContent = current.observation_time;
document.getElementById("temperature").textContent = `${current.temperature}°C`;
document.getElementById("weather_description").textContent = current.weather_descriptions[0];
document.getElementById("location").textContent = data.location.name;
// Fill in historical data
const historicalData = data.historical;
const mainContent = document.querySelector(".main-content");
for (const date in historicalData) {
const historicalInfo = historicalData[date];
const dayOfWeek = new Date(date).toLocaleDateString('en-US', { weekday: 'short' });
const weatherColumn = document.createElement("article");
weatherColumn.classList.add("weather-column");
weatherColumn.innerHTML = `
<span class="weather-symbol"></span>
<p class="day">${dayOfWeek}</p>
<p class="temperature">${historicalInfo.avgtemp}°</p>
`;
mainContent.appendChild(weatherColumn);
}
// Fill in current date
const dateParts = data.location.localtime.split(' ')[0]
const formattedDate = `${dateParts[2]}-${dateParts[1]}-${dateParts[0]}`;
const dayOfWeek = new Date(dateParts).toLocaleDateString('en-US', { weekday: 'short' });
const weatherColumn = document.createElement("article");
weatherColumn.classList.add("weather-column");
weatherColumn.classList.add("is-active");
weatherColumn.innerHTML = `
<span class="weather-symbol"></span>
<p class="day">${dayOfWeek}</p>
<p class="temperature">${current.temperature}°</p>
`;
mainContent.appendChild(weatherColumn);
})
.catch(error => console.error("Error:", error));
</script>
</body>
</html>
With this application, we will use the historical endpoint of the weatherstack API with the date range. The weatherstack API also provides current weather data at the historical weather data endpoint. For this, we added the days before today while specifying the date range in the weatherstack API. Before running this application, let’s replace ‘YOUR-API-KEY’ with our own API key and run the application.
The historical weather dashboard we get after running the application is as follows:
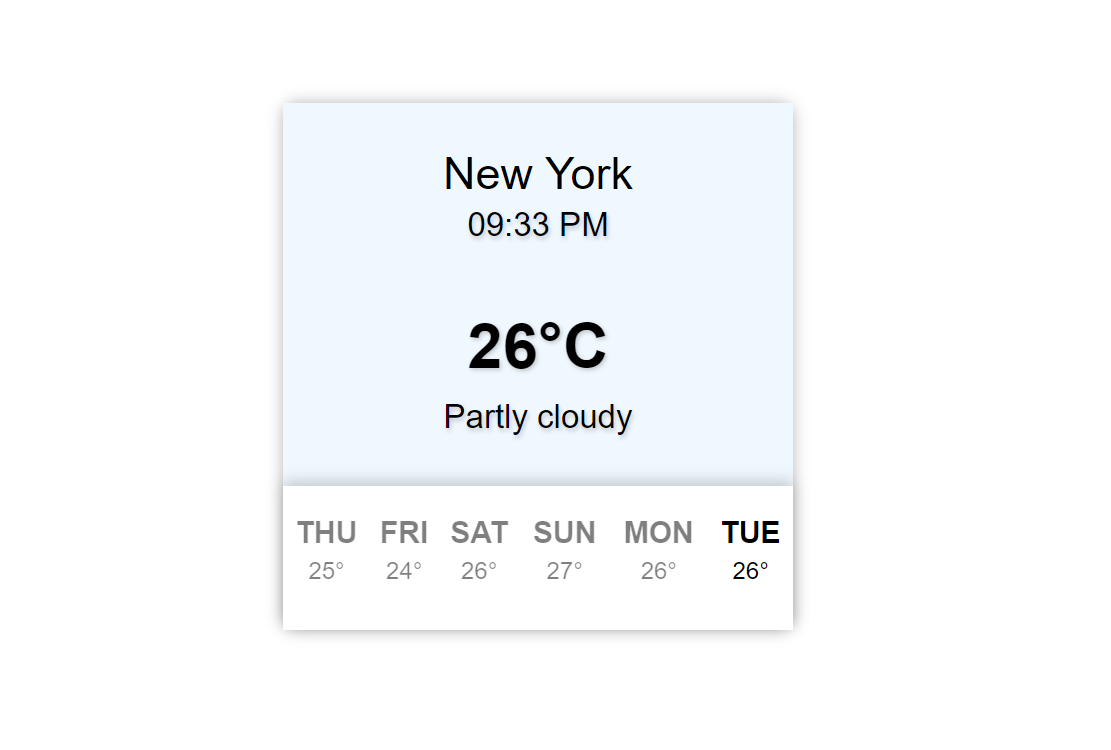
Conclusion
To sum up, historical data can be used in a wide range from climate change analysis to agricultural planning, from energy management to construction projects, and offers businesses a valuable perspective on their decision-making processes. Creating a functional and information-filled weather dashboard by effectively using weather data to increase user experience, especially for many platforms, helps users make better decisions.
Check out our API providing historical weather data, quickly get historical data up to 2008!
FAQs
Q: Is the weatherstack a Historical Weather API?
A: Yes, it is. The weatherstack API is a very powerful API that provides both historical and current weather data for millions of locations. It also provides weather forecast data.
Q: What are the Popular Historical Weather APIs?
A: Some of the popular historical weather APIs in the market are as follows:
- The weatherstack API
- OpenWeatherMap API
- Aeris Weather API
- Tomorrow.io API
Q: Does the weatherstack Historical Weather API Get the Data It Provides from Official Weather Stations?
A: Yes, it does. The weatherstack API receives weather data from the weather station, making it a reliable and accurate weather API.
Q: Does the weatherstack API Provide Wind Speed Information?
A: Yes, it does. It also provides wind direction and wind degree.
Read: How to Create a Great Weather Dashboard With a Weather API – Part 1 (Current Weather)
Read: How to Create a Great Weather Dashboard With a Weather API – Part 3 (Weather Forecast)
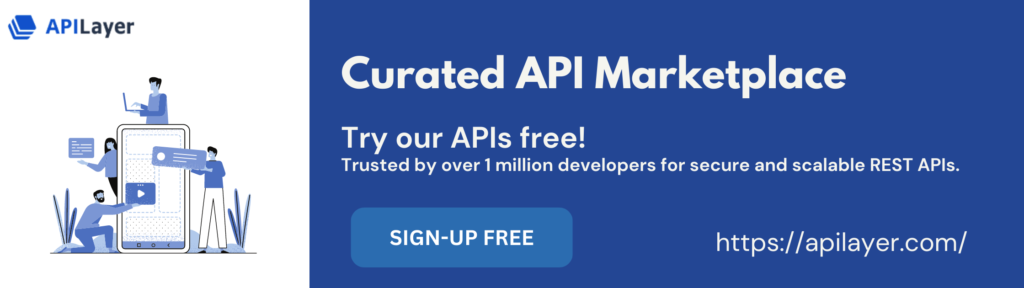